ReactElement: Building a todo web application
Objective
In this project, students will learn the core steps of React, including creating React components, managing state, and handling user interactions. They will gain hands-on experience in these fundamental React concepts and techniques.
Learning Outcomes
By the end of this project, learners will be able to build a simple React application. Learners will be able to:
- Set up a new React application using Create React App.
- Create functional and class components in React.
- Manage state and handle user interactions using React hooks and class methods.
- Utilize props to pass data between components.
- Style the application using CSS or a CSS framework.
Steps and Tasks
Set up a new React application using Create React App
-
Install Create React App globally
npm install -g create-react-app
-
Create a new React project:
npx create-react-app my-app-name
This creates a folder named my-app-name where all the files reside -
Install additional libraries as needed
npm install react-bootstrap bootstrap
npm install @mui/material @emotion/react @emotion/styled
Create a functional component for a basic layout
- In the
src
folder, create a new file calledLayout.js
. - Define a functional component that renders a basic layout with a header, main content, and footer. Import Layout.js into your main app.
Sample layout file
import React from 'react';
import Header from './Header';
import MainContent from './MainContent';
const Layout = () => {
const title = 'My Todo App';
const tasks = ['Task 1', 'Task 2', 'Task 3'];
return (
<div>
<Header title={title} />
<MainContent tasks={tasks} />
<footer>
<p>Footer</p>
</footer>
</div>
);
};
export default Layout;
Build class components to manage state and handle user interactions
-
Create a new file called TodoApp.js in the src folder of your React project.
-
Inside TodoApp.js, import the necessary dependencies:
import React, { Component } from 'react';
-
Define a class component called TodoApp that extends the Component class
-
Inside the TodoApp component, define a constructor method to initialize the component’s state
-
Define methods for handling user interactions and updating the state:
- handleChange: This method will handle changes in the new task input. It should update the newTask state with the current value of the input.
- handleSubmit: This method will handle the form submission when the user adds a new task. It should prevent the default form submission behavior, check if the new task input is not empty, and update the tasks state with the new task.
- handleDelete: This method will handle the deletion of a task. It should remove the task from the tasks state array based on its index.
-
Implement the render method to define the UI of the todo list component
- Render an input field for adding new tasks, a submit button, and a list of tasks.
- Map over the tasks state array to render each task with a delete button.
- Attach the appropriate event handlers to the input field, form, and delete button.
-
Export the TodoApp component as the default export from TodoApp.js:
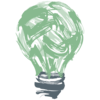
Utilize props to pass data between components
-
Pass a prop called title to the Layout component from the App component, and assign it a value of your choice.
-
Pass a prop called tasks to the Layout component from the App component, and assign it an array of tasks that you want to display in the main content area.
Style the application using CSS or a CSS framework
- Use CSS or a CSS framework like Bootstrap or Material-UI.
- Create a new CSS file called
styles.css
in thesrc
folder. - Add styles to customize the appearance of the header, main content, and footer components.
- Import the
styles.css
file into your components and apply the styles as needed. - Import additional components as desired
import { Button, Card, Form } from 'react-bootstrap';
Sample css file
.header {
background-color: #f8f9fa;
padding: 20px;
text-align: center;
}
.main-content {
margin: 20px;
}
.footer {
background-color: #f8f9fa;
padding: 20px;
text-align: center;
}
Evaluation
This starter project is not being used for virtual internship evaluations. However, learners can use the following points for self-evaluation and to ensure they have achieved a growth mindset in their React development skills!
-
Comfort with React: Learners should feel comfortable working with React and have a solid understanding of its core concepts and principles.
-
Building Interactive UI: Learners should be able to build interactive user interfaces using React components and handle user interactions effectively.
-
State Management: Learners should have a good grasp of state management in React, whether using hooks or class components, and be able to handle state changes and updates efficiently.
-
Component Reusability: Learners should be able to create reusable components and effectively use props to pass data between components for better code organization and maintainability.
-
CSS Styling: Learners should have the ability to style their React applications using CSS or CSS frameworks, ensuring a visually appealing and responsive user interface.
-
Exploration of Advanced Concepts: Learners should feel encouraged to explore more advanced React concepts and libraries beyond the project, such as React Router for routing or integrating external APIs for data retrieval and manipulation.
Resources and Learning Materials
- React: https://reactjs.org/docs/getting-started.html
- Create React App: https://create-react-app.dev/
- FreeCodeCamp’s React course: https://www.freecodecamp.org/learn/front-end-libraries/react/
- Node.js: https://nodejs.org/
- Visual Studio Code: https://code.visualstudio.com/
- CSS Framework: Bootstrap (https://getbootstrap.com/) or Material-UI (https://mui.com/)
Need a little extra help?
If you are having trouble getting started, click below to see some skeleton code. This code includes the necessary class structure, constructor method, event handlers, and render method for the TodoApp
component.
You can take this sample code as a starting point and further enhance the to-do list app by adding features like marking tasks as complete, filtering tasks, adding due dates, or using local storage for persistent data. We encourage you to experiment and explore different aspects of React to create your own unique version of the to-do list app.
Click to see skeleton code
import React, { Component } from 'react';
class TodoApp extends Component {
constructor() {
super();
this.state = {
tasks: [],
newTask: '',
};
}
handleChange = (event) => {
this.setState({ newTask: event.target.value });
};
handleSubmit = (event) => {
event.preventDefault();
const { tasks, newTask } = this.state;
if (newTask.trim() !== '') {
const updatedTasks = [...tasks, newTask];
this.setState({ tasks: updatedTasks, newTask: '' });
}
};
handleDelete = (index) => {
const { tasks } = this.state;
const updatedTasks = [...tasks.slice(0, index), ...tasks.slice(index + 1)];
this.setState({ tasks: updatedTasks });
};
render() {
const { tasks, newTask } = this.state;
return (
<div>
<h2>Todo List</h2>
<form onSubmit={this.handleSubmit}>
<input
type="text"
value={newTask}
onChange={this.handleChange}
placeholder="Add a new task"
/>
<button type="submit">Add</button>
</form>
<ul>
{tasks.map((task, index) => (
<li key={index}>
{task}
<button onClick={() => this.handleDelete(index)}>Delete</button>
</li>
))}
</ul>
</div>
);
}
}
export default TodoApp;